Automation Testing (Registration Form)
In this post, I want to share how to automate a simple HTML registration form using Selenium python. Before starting that, let’s look briefly at automation testing and why we should use it, its advantages over manual testing, and questions being asked about automation testing.
According to Wikipedia, Software testing is an investigation conducted to provide stakeholders with information about the quality of the software product or service under test. In my words, software testing is a method to check if our product performs as expected based on the requirements.
The purpose of software testing is to find any defect in our product or any gaps in the flow of the product’s functionality.
There are two methods to perform software testing.
- Manual Testing: Manual testing means we need to run our test cases manually one by one, which can sometimes be very tedious and time-consuming work.
- Automation Testing: Automation testing means performing and executing our testing using some available software automation tools like Selenium.
It is advisable that the regression test suite should be automated as automation saves time, effort, and cost.
In the software testing world, in the case of testing RESTFUL API tests and web UI tests people are moving more towards automation. To automate test suites for APIs and web application UI, there are various tools available and can be used directly without doing the complicated setup or without having prior knowledge of coding.
But my personal opinion is that not everything can be automated. it’s a balance between the time and money you want to invest to achieve the desired quality using automation or manual testing
In this post, I am going to talk about automation Testing.
The advantage of automation testing is:
- The test execution is very fast, so it saves a lot of time in testing.
- It saves human effort.
- There is less chance of error.
- It is beneficial in the case of repeated tests.
- More efficient if properly designed. e.g., saves log, other data for analysis.
There are so many tools available for automation testing like Selenium and katalon studio. The most widely used for UI automation is Selenium. Selenium is an open-source framework for automation that can be used to validate the web-applications. It is compatible with multiple languages like Java, C#, Python, etc.
For my post, I will use the Selenium Framework and write a script using python language. We can divide the steps for automation using Selenium as:
- Create a web browser instance like chrome, firefox, etc
- Invoke the web browser and navigate to the webpage
- Find a unique HTML element based on its attribute
- Perform an action like entering the text from the keyboard or clicking the radio button.
- Run the test and record the response of the action performed on the webpage
- Conclude the test.
I am going to write a simple python code using the Selenium framework to automate a webpage I have created.
https://mytestingthoughts.com/Sample/home.html
Let’s see this simple example of a Registration form, I am going to write a Selenium script for this form.
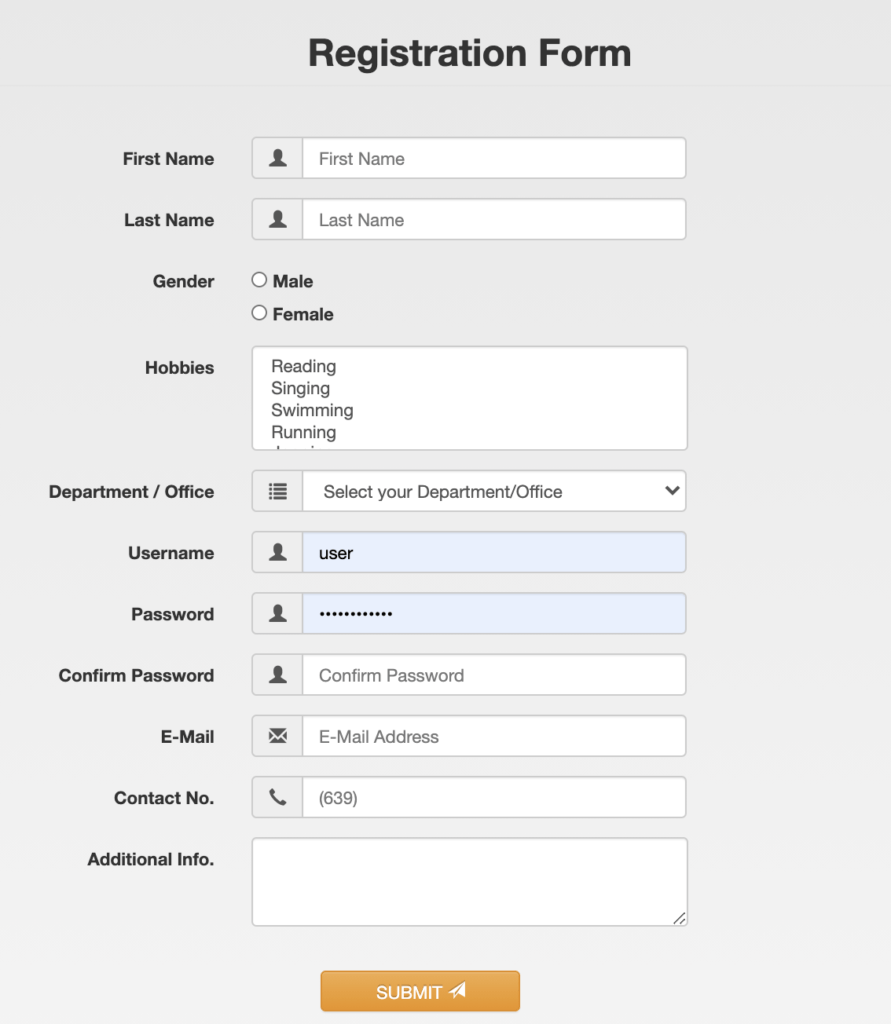
from selenium import webdriver from selenium.webdriver.common.keys import Keys import time import traceback from selenium.webdriver.support.ui import Select from selenium.common.exceptions import * if __name__ == '__main__': try: driver = webdriver.Chrome("/Webdriver path") driver.get("https://mytestingthoughts.com/Sample/home.html") driver.maximize_window() # First_name f_name = driver.find_element_by_name("first_name") f_name.send_keys("Abcdef") # Last_name l_name = driver.find_element_by_name("last_name") l_name.send_keys("xyz") # Gender male = driver.find_element_by_name('inlineRadioOptions').click() # Hobbies hobbies = driver.find_element_by_id("exampleFormControlSelect2") select = Select(hobbies) select.select_by_visible_text("Reading") # Department/office department = driver.find_element_by_name("department") select = Select(department) select.select_by_visible_text("Department of Engineering") # username user_name = driver.find_element_by_name("user_name") user_name.send_keys("user1234") # password pwd = driver.find_element_by_name("user_password") pwd.send_keys("abc_1234") # Confirm_password con_pwd = driver.find_element_by_name("confirm_password") con_pwd.send_keys("abc_1234") # E-mail email = driver.find_element_by_name("email") email.send_keys("abcdef.xyz@gmail.com") # Contact-no cont_no = driver.find_element_by_name("contact_no") cont_no.send_keys("123-456-7890") # AdditionalInfo ad_info = driver.find_element_by_id("exampleFormControlTextarea1") ad_info.send_keys("Abc is a good person") # Submit # submit_button = driver.find_element_by_class_name("btn-warning") submit_button = driver.find_element_by_xpath("//button[@type='submit']") submit_button.click() html = driver.find_element_by_tag_name('html') html.send_keys(Keys.END) except (NoSuchElementException,ElementNotVisibleException,ElementNotSelectableException) as e: print(str(e)) except Exception as e: print("Some error occured"+ str(e)) traceback.print_exc() finally: time.sleep(3) driver.quit()
Now let us see how we locate an element on the web app UI:
Locate an element on the web app UI
To automate the UI testing using Selenium, it is crucial to understand how to locate any element on the DOM (logical structure of HTML of the web page). To perform any action/operation on the web app UI, we need to tell Selenium on which element we want to perform any action. There are tools available as an extension to the browsers to find out any element’s location. Still, it is always better to understand the concept of finding elements on web UI. This practice makes coding in Selenium more fun and logical.
There are many ways to find an element on a web UI page, as mentioned below:
- By Id
- By Class name
- By Xpath
- By Link text
- By Partial link text
- By Name
- By CSS selector
- By Tag name
The most common way of finding an element is XPath. The goal is to find the unique property of the element by which we can identify the element. Here I’m going to explain how we can find the elements using a unique id/class name and XPath. For example, below is a snippet of a DOM of a standard web app.
!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Bootstrap Template</title> </head> <body> <div class="container"> <form class="well form-horizontal" action=" " method="post" id="contact_form"> <div class="form-group"> <label class="col-md-4 control-label">First Name</label> <div class="col-md-4 inputGroupContainer"> <div class="input-group"> <span class="input-group-addon"><i class="glyphicon glyphicon-user"></i></span> <input name="first_name" placeholder="First Name" class="form-control" type="text"> </div> </div> </div> <div class="alert alert-success" role="alert" id="success_message">Success <i class="glyphicon glyphicon-thumbs-up"></i> Success!.</div> </form> </div> </body> </html>
Steps to find an element
- Open the web application UI you want to automate.
- Right-click and select Inspect.
- A dev tool window will open up, showing details of the page.
- The tab Elements should be selected by default; if not, select the tab Elements.
- The above-shown example like DOM will be available.
- There is a mouse icon available at the left corner; select that icon.
- Hover on any element on the web page elements, and the relevant section in the DOM will be highlighted.
- Click the element you want to find the location.
- Analyze the unique property as explained below and get the location.
Find the element’s location on the DOM by Id/name/class name/tag name/link text.
Read the line selected on the DOM carefully, if it has id/name/class name/tag name/link etc which is unique on the page then save that unique identifier Id/name/class name/tag name/link text. Through that id, we can operate on the element through Selenium
<div class="alert alert-success" role="alert" id="success_message">Success <i class="glyphicon glyphicon-thumbs-up"></i> Success!.</div>
In the above line, there is an id success_message mentioned. Copy the id and find it in the DOM, if it is used only once that’s the id we are looking for.
Now that we have a unique identifier, we can use a similar method like find_element_by_id in python to get the driver object of Selenium’s element.
driver.find_element_id('success_message')
– Don’t worry, we’ll understand the details of writing the code later.
Find the location of the element on the DOM by Xpath.
It sometimes happens that an element doesn’t have id/class_name etc., or doesn’t have them uniquely. In that case, we can use the XPath of the element to identify it.
There are two types of XPath:
- Absolute
- Relative
Absolute – The absolute path is finding the element by traversing over all the elements from the beginning and reaching that element. It starts with a single /
- In above-provided example, if you have to find the element <div> which has class=”alert alert-success” line21.
/html/body/div/form/div[2]
To get to line 14
/html/body/div/form/div/div
Relative – The relative path is finding the unique identifier in the closest element and then traversing over the remaining path. It starts with double //
- In above-provided example, if you have to find the element <div> which has class=”alert alert-success” line21.
//div[@id='success_message']
To get to line 14
//div[@class='form-group']/div[1]
Relative XPath is faster than absolute XPath.
Find the XPath by using Chrome/Firefox.
- Right-click on the element.
- Select Copy.
- For relative XPath, select Copy Xpath.
- For absolute XPath, select Copy full Xpath.
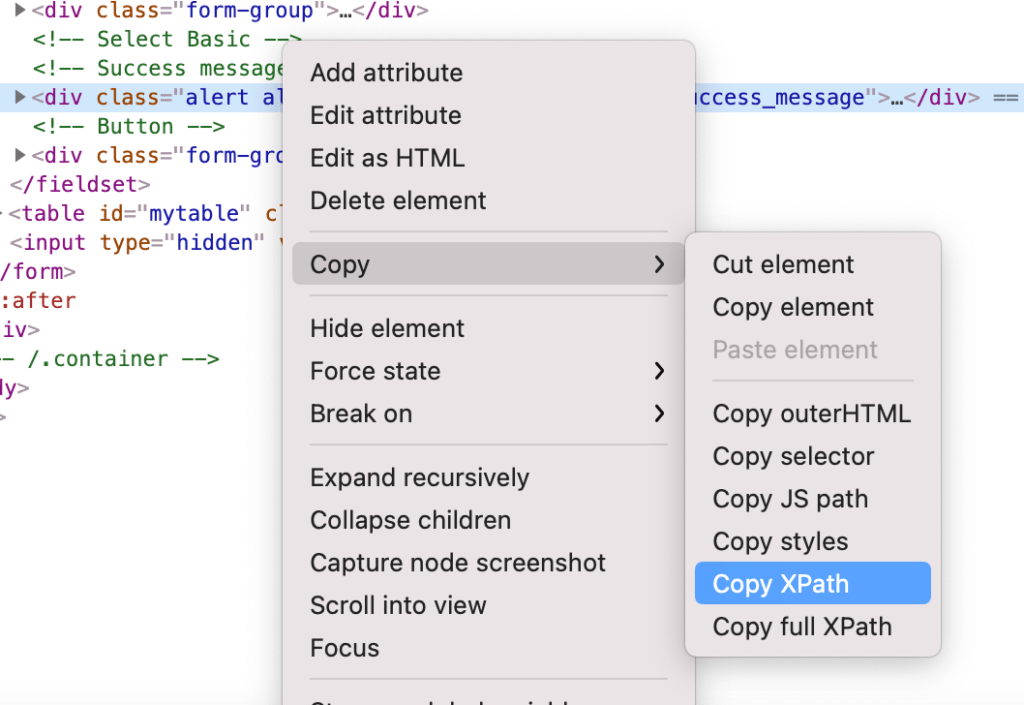
After finding the element’s XPath we can use the method find_element_by_xpath in Python using the Selenium framework to operate on the element.
e.g.
driver.find_element_by_xpath('//div[@id='success_message']).click